Wifi Propagation
Summary
In this tutorial, we will study the wifi signal power in a flat. An awesome flat is especially designed for the experiment, with 2 walls:
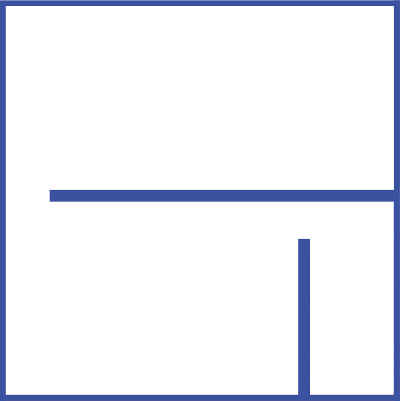
Even if the flat seems small enough to be covered by wifi everywhere, it is still interesting to study where the signal’s power is the lowest. We will study where to put the hotspot to get the best coverage, and as we’re a bit lazy we will only put it next to the left wall.
Physics
In a nutshell, the Wifi is a electromagnetic wave that contains a signal : Internet data. Electromagnetic waves are well know by physicists and are ruled by the 4 Maxwell equations which give you the solution for E, the electrical field, and B, the magnetic field, in space but also in time.
We don’t care about the time here, because the signal period is really short so our internet quality will not change with time. Without time, we’re looking for stationaries solutions, and the Maxwell equations can be simplified to one equation, the Helmholtz one :
Where k is the angular wavenumber of the wifi signal, and n the refractive index of the material the wave is in.
Indeed, the main point of this study is the impact of walls on the signal’s power, where the n is different from air (where it is 1). In walls, the refractive index is a complex number in which the two parts have a physic interpretation:
The real part defines the reflexion of the wall (the amount of signal that doesn’t pass).
The imaginary part defines the absorption of the wall (the amount that disappears).
The wifi hotspot (simulated by a simple circle) will be the boundary condition, with a non null value for our electrical field.
Coding
The domain
In order to create the domain of experimentation, we need to create border
objects, like this :
1real a = 40, b = 40, c = 0.5;
2border a00(t=0, 1) {x=a*t; y=0; label=1;}
3border a10(t=0, 1) {x=a; y=b*t; label=1;}
4border a20(t=1, 0) {x=a*t; y=b; label=1;}
5border a30(t=1, 0) {x=0; y=b*t; label=1;}
6border a01(t=0, 1) {x=c+(a-c*2)*t; y=c; label=1;}
7border a11(t=0, 1) {x=a-c; y=c+(b-c*2)*t; label=1;}
8border a21(t=1, 0) {x=c+(a-c*2)*t; y=b-c; label=1;}
9border a31(t=1, 0) {x=c; y=c+(b-c*2)*t; label=1;}
10
11real p = 5, q = 20, d = 34, e = 1;
12border b00(t=0, 1) {x=p+d*t; y=q; label=3;}
13border b10(t=0, 1) {x=p+d; y=q+e*t; label=3;}
14border b20(t=1, 0) {x=p+d*t; y=q+e; label=3;}
15border b30(t=1, 0) {x=p; y=q+e*t; label=3;}
16
17real r = 30, s =1 , j = 1, u = 15;
18border c00(t=0, 1) {x=r+j*t; y=s; label=3;}
19border c10(t=0, 1) {x=r+j; y=s+u*t; label=3;}
20border c20(t=1, 0) {x=r+j*t; y=s+u; label=3;}
21border c30(t=1, 0) {x=r; y=s+u*t; label=3;}
Let’s create a mesh
1int n=13;
2mesh Sh = buildmesh(a00(10*n) + a10(10*n) + a20(10*n) + a30(10*n)
3 + a01(10*n) + a11(10*n) + a21(10*n) + a31(10*n)
4 + b00(5*n) + b10(5*n) + b20(5*n) + b30(5*n)
5 + c00(5*n) + c10(5*n) + c20(5*n) + c30(5*n));
6plot(Sh, wait=1);
So we are creating a mesh
, and plotting it :
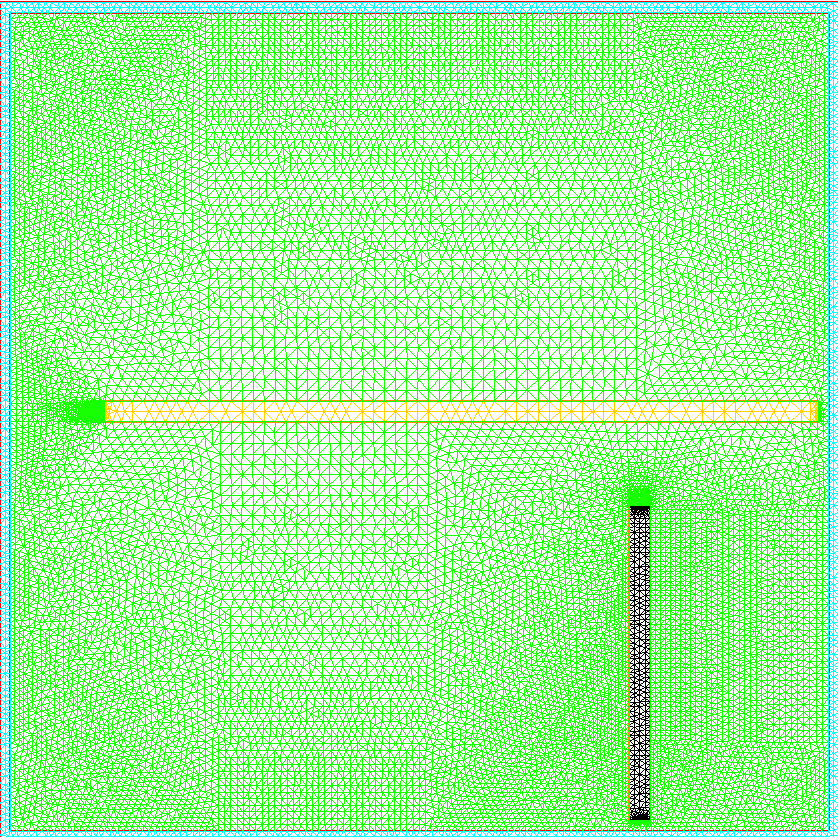
There is currently no wifi hotspot, and as we want to resolve the equation for a multiple number of position next to the left wall, let’s do a for
loop:
1int bx;
2for (bx = 1; bx <= 7; bx++){
3 border C(t=0, 2*pi){x=2+cos(t); y=bx*5+sin(t); label=2;}
4
5 mesh Th = buildmesh(a00(10*n) + a10(10*n) + a20(10*n) + a30(10*n)
6 + a01(10*n) + a11(10*n) + a21(10*n) + a31(10*n) + C(10)
7 + b00(5*n) + b10(5*n) + b20(5*n) + b30(5*n)
8 + c00(5*n) + c10(5*n) + c20(5*n) + c30(5*n));
The border C
is our hotspot and as you can see a simple circle.
Th
is our final mesh, with all borders and the hotspot.
Let’s resolve this equation !
1fespace Vh(Th, P1);
2func real wall() {
3 if (Th(x,y).region == Th(0.5,0.5).region || Th(x,y).region == Th(7,20.5).region || Th(x,y).region == Th(30.5,2).region) { return 1; }
4 else { return 0; }
5}
6
7Vh<complex> v,w;
8
9randinit(900);
10Vh wallreflexion = randreal1();
11Vh<complex> wallabsorption = randreal1()*0.5i;
12Vh k = 6;
13
14cout << "Reflexion of walls min/max: " << wallreflexion[].min << " " << wallreflexion[].max << "\n";
15cout << "Absorption of walls min/max: " << wallabsorption[].min << " "<< wallabsorption[].max << "\n";
16
17problem muwave(v,w) =
18 int2d(Th)(
19 (v*w*k^2)/(1+(wallreflexion+wallabsorption)*wall())^2
20 - (dx(v)*dx(w)+dy(v)*dy(w))
21 )
22 + on(2, v=1)
23 ;
24
25muwave;
26Vh vm = log(real(v)^2 + imag(v)^2);
27plot(vm, wait=1, fill=true, value=0, nbiso=65);
28}
A bit of understanding here :
The
fespace
keyword defines a finite elements space, no need to know more here.The function
wall
return 0 if in air and 1 if in a wall (x and y are global variables).For this example, random numbers are used for the reflexion and the absorption.
The problem is defined with
problem
and we solve it by calling it.
Finally, I plotted the \(\log\) of the module of the solution v
to see the signal’s power, and here we are :
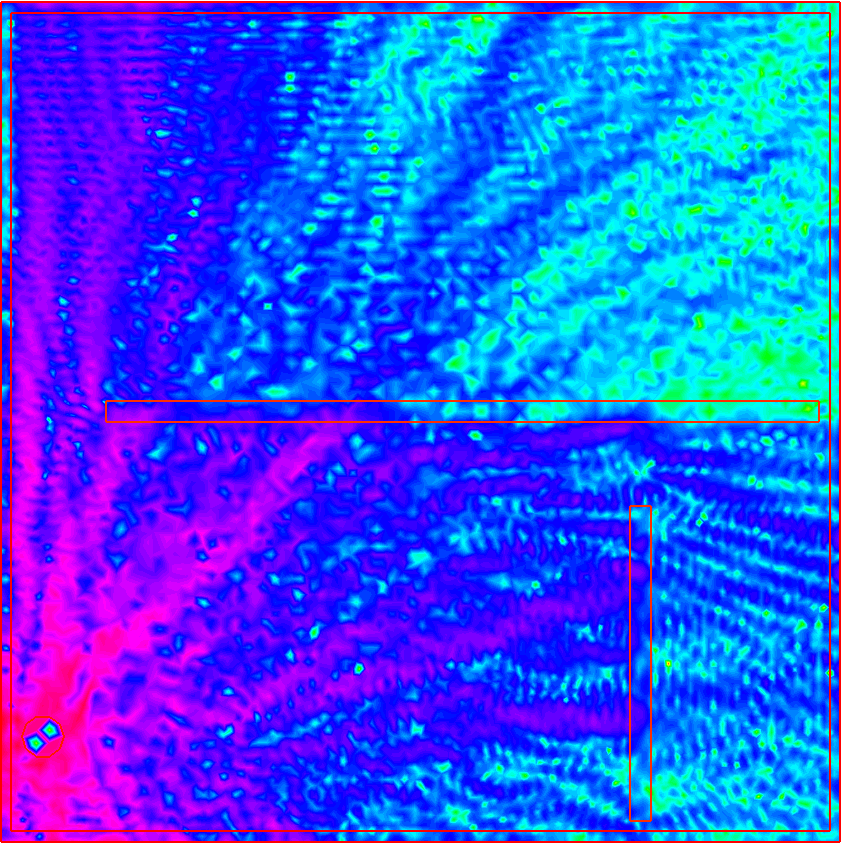
Beautiful isn’t it ? This is the first position for the hotspot, but there are 6 others, and the electrical field is evolving depending on the position. You can see the other positions here :